Integrations.js Overview
Integrations.js documentation and quickstart.
Integrations.js makes it dead simple to add integrations to your site without having to build, test, and implement them from scratch each time.
The library is open source, and you can view integrations.js on Github.
Supported Integrations
As of today, integrations.js supports the following integrations out of the box:
- Google Sheets: let users sign in with Google, and export data from your page into Sheets.
- Trello: let users sign in with Trello, and create cards given data on your page.
- Clearbit: let users sign in with Clearbit, and lookup profile information about people mentioned on your page.
Getting Started
This tutorial will help you start adding integrations to your site using the Integrations.js library. As soon as you’re setup you’ll be able to turn on any new integration with the flip of a switch!
Step 1: Choose Integrations
Installing Integrations.js is easy. First, visit your Integrations.js dashboard and toggle the integrations you want activated on your site.
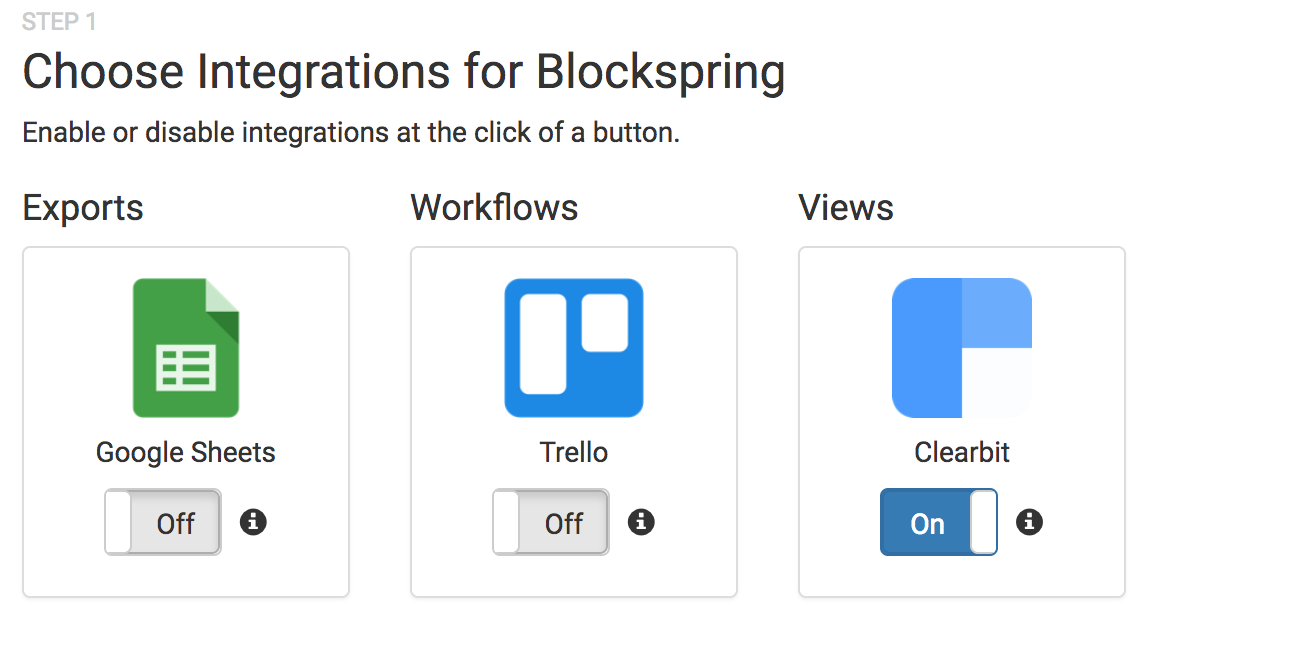
Step 2: Copy the Snippet
Now you can copy/paste the Integrations.js library and JS snippet into your site, wherever you'd like to see the integrations. Here's a sample code snippet.
<script>
(function(){
var integrations=window.integrations=window.integrations||[];if(integrations.loaded){return}if(integrations.invoked){return}integrations.invoked=true;integrations.methods=["identify","setContext","set","get","do"];integrations.factory=function(method){return function(){var args=Array.prototype.slice.call(arguments);args.unshift(method);integrations.push(args);return integrations}};for(var i=0;i<integrations.methods.length;i++){var key=integrations.methods[i];integrations[key]=integrations.factory(key)}integrations.load=function(siteId){integrations.siteId=siteId;var script=document.createElement("script");script.type="text/javascript";script.async=true;script.src="https://cdn.integrationsjs.com/dist/boot.js";var first=document.getElementsByTagName("script")[0];first.parentNode.insertBefore(script,first)};
// Load Integrations.js with your API key
integrations.load('API_KEY_HERE');
// Identify the user by their unique ID
integrations.identify({
userId: 'UNIQUE ID FOR USER' // email/username/userid
});
// Call setContext whenever the context of the page changes
// This can be called any number of times
integrations.setContext({
people: [{
email: 'jason@blockspring.com',
name: 'Jason Tokoph'
}],
title: 'So many integrations, so little time',
description: 'Take a look at integrationsjs.com',
datasets: {
foo: [['name', 'age'], ['jason', 29]]
}
});
})();
</script>
If you enabled Clearbit, and pasted this code snippet into your site with the proper API token in your account, then you've completed your first integration. You should see Clearbit enabled on your site.
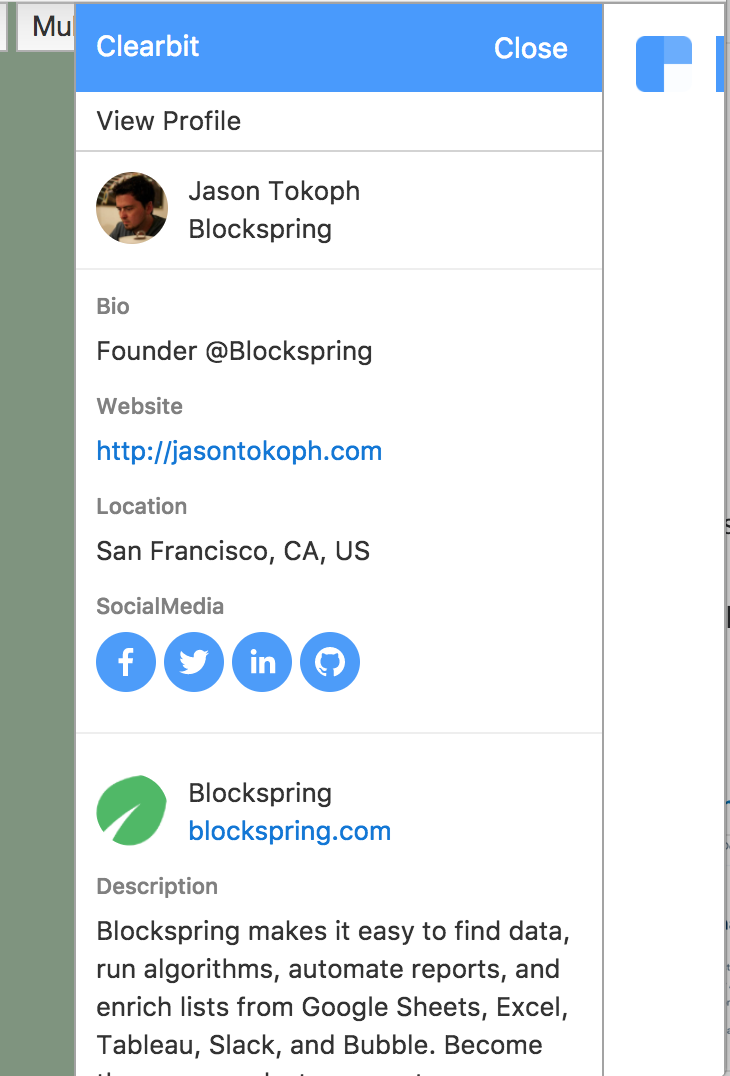
Context
You can power integrations by passing data into the integrations.setContext
method.
Export integrations
Export integrations let users export data from your site into apps like Google Sheets. The typical usecase is for reporting.
These integrations require you to pass a datasets
context. You can pass multiple tables to export into the datasets
context. Each table must be an array of arrays, and a named key.
The below example shows an export integration with a single 'invoices' dataset:
integrations.setContext({
datasets: {
invoices: [
['name', 'bill'],
['jason', 29],
['christy', 12]
]
}
});
Workflow integrations
Workflow integrations let users complete workflows without leaving your site - like creating a Trello card using data from your page.
These integrations require you to pass a title
and description
context. For instance, if you're using the Trello integration it'll prefill the 'create a card' page with the title and description you provide.
The below example shows a workflow integration context:
integrations.setContext({
title: 'So many integrations, so little time',
description: 'Take a look at integrationsjs.com',
});
View integrations
View integrations let users access to their own data (or 3rd party) from your site - like seeing a user's profile from a CRM or internal system.
These integrations require you to pass a people
context. You can pass multiple people, as long as each is an object with an 'email' key.
The below example shows a view integration context:
integrations.setContext({
people: [{
email: 'jason@blockspring.com'
}, {
email: 'willis@example.com'
}],
});
Updated less than a minute ago