Tutorial: App Connectors and Buttons
Let's learn how to use app connectors, and buttons to initiate scripts.
Usecase: Click to Sync with Google Sheets
Spreadsheets are great for backing up information, as well as updating information en masse. In this tutorial, we'll write a script that exports Trello cards to Google Sheets, then lets us edit a bunch of cards at once from a sheet, and import back into Trello.
ENABLE A CONNECTOR
Usually, integrating with another app requires setting up OAuth or getting an API token. Blockspring Scripts handles this so you can choose your connector, sign in, and get started with your integration.
- Click Add+ next to Connectors in the right sidebar.
- Choose your connector, in this case Google Sheets .
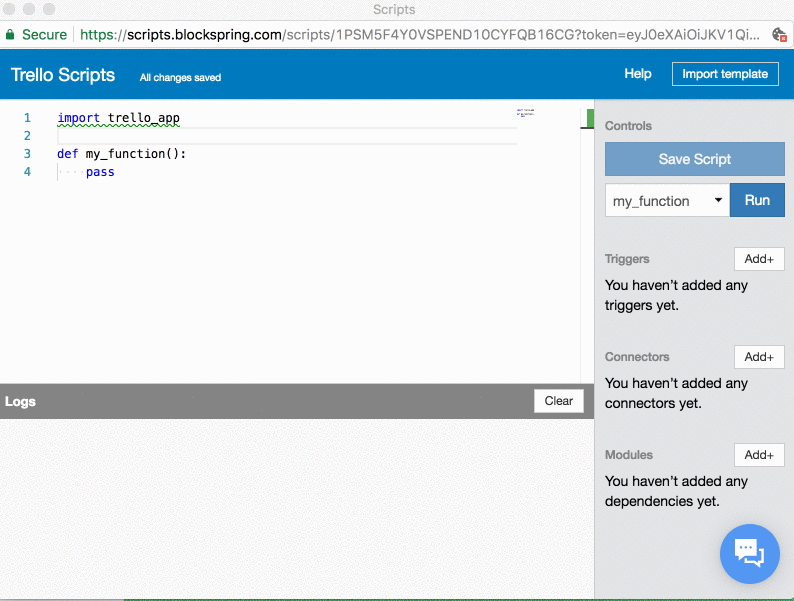
You'll notice the editor automatically handles OAuth/authentication with Google, and imports google_sheets_app
. You're now able to script with Sheets, just like you do with Trello.
EXPORT CARDS TO SHEET
Enter in the following code to export all your open cards to Google Sheets.
import google_sheets_app
import trello_app
## STEP 1: SIGN IN WITH GOOGLE SHEETS IN THE SIDEBAR
## STEP 2: PASTE A GOOGLE SHEET'S ID BELOW (FOUND IN ITS URL)
sheet_id = "PASTE_YOUR_SHEET_ID_HERE"
def export_to_sheets():
# get all open cards in this board
board = trello_app.get_current_board()
cards = board.get_cards(filter="open")
# export cards to the google sheet
sheet = google_sheets_app.get_spreadsheet_by_id(sheet_id).get_sheets()[0]
sheet.write_values(cards)
Since we've already signed in with Google Sheets, the next step is to find a sheet ID. You'll want to do the following:
- Open a new or existing Google Sheet.
- Save the sheet and make sure it has a name.
- Copy the sheet ID, found in its URL, into your script.
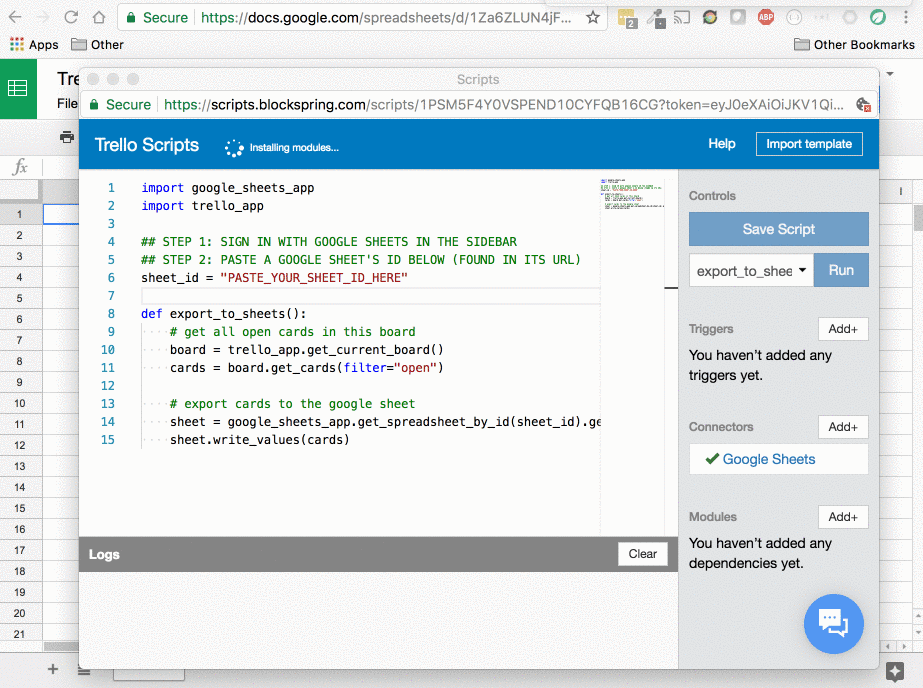
Finally, let's run our export function. However, instead of using the test button in the editor, let's go back to Trello.
- Hover over the Blockspring leaf on your Trello board,
- Click the
export_to_sheets
menu option.
Check out the sheet - it has our cards!
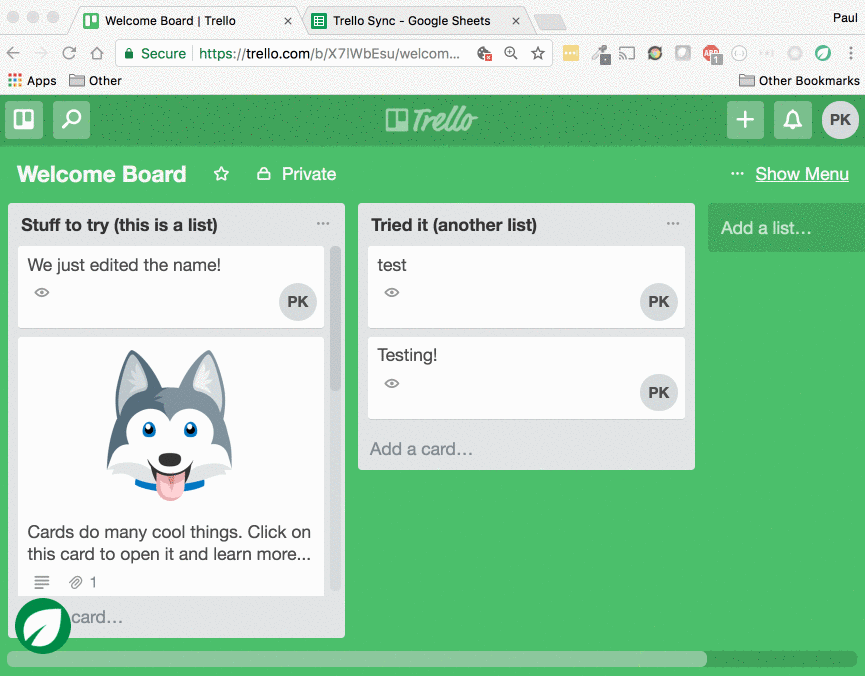
Running scripts from the Blockspring leaf
Functions you add to your script are all available to run from the Blockspring leaf, outside of the script editor.
Go to the board (or Asana project, FIeldbook book, etc) associated with your script, and instead of clicking the leaf to open the editor, just hover over the leaf and click the function you want to run it.
IMPORT CARDS FROM SHEET
Adding the ability to edit your cards in the Google Sheet and then importing is as easy as updating your function with the following code.
Don't forget to paste in your Sheet ID here as well.
import google_sheets_app
import trello_app
## STEP 1: SIGN IN WITH GOOGLE SHEETS IN THE SIDEBAR
## STEP 2: PASTE A GOOGLE SHEET'S ID BELOW (FOUND IN ITS URL)
sheet_id = "PASTE_YOUR_SHEET_ID_HERE"
def export_to_sheets():
# get all open cards in this board
board = trello_app.get_current_board()
cards = board.get_cards(filter="open")
# export cards to the google sheet
sheet = google_sheets_app.get_spreadsheet_by_id(sheet_id).get_sheets()[0]
sheet.write_values(cards)
def import_from_sheets():
# read the google sheet
sheet = google_sheets_app.get_spreadsheet_by_id(sheet_id).get_sheets()[0]
sheet_data = sheet.get_values()
# for each row in sheet
for row in sheet_data:
# look for an ID column, and lookup that card
card = trello_app.get_card_by_id(row.get("id"))
# if you find a card, update it with the row
if card:
card.set_field(row)
# if this card doesn't exist, create it
else:
li = trello_app.get_list_by_id(row.get("idList"))
li.create_card(row)
# when done, pull updated card list into sheets
export_to_sheets()
Let's walk through how this code works:
- First, we get values from our spreadsheet's first sheet.
- Next, we go through each row attempting to look up the card in Trello give the "id" column.
- If a card exists, we use
.set_field()
to update it with whatever updated information we have in our spreadsheet. - If a card does not exist, we create it in using the "idList" column.
- Finally, once all cards are updated or created, we run
export_to_sheets
to update our spreadsheet with the latest card list from Trello.
Here's a GIF of what this look like when run from the Blockspring leaf on Trello.
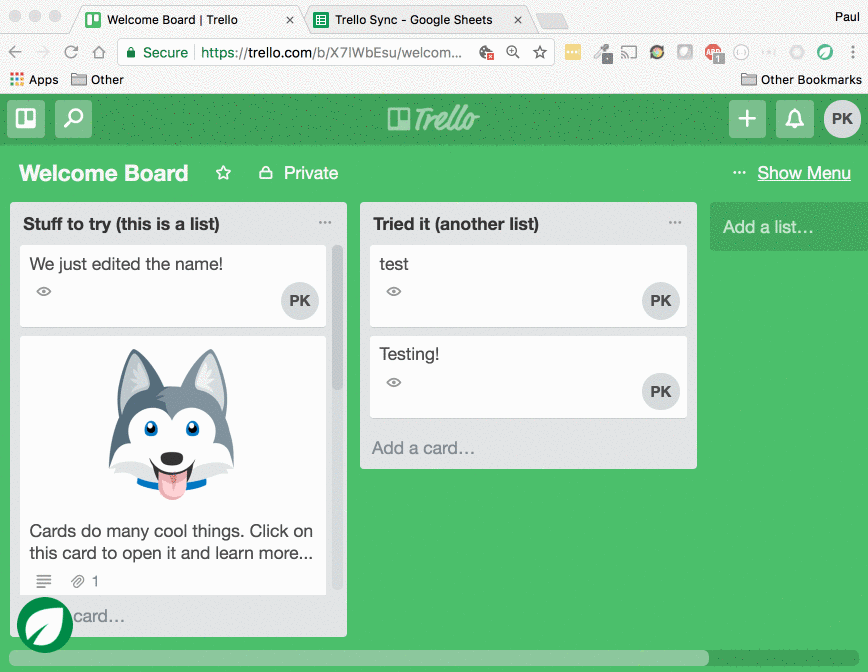
Need Help?
If you run into any issues, or what to chat with others, connect to the Blockspring community right from the Scripts editor.
Updated less than a minute ago