Tutorial: Custom Reports and Modules
Let's learn about how to create custom reports, and use Python modules.
Usecase: Email Trello Card Assignment Report
Most apps don't provide exactly the right report you might need for yourself, or to share with your team. In this tutorial, we'll write a script that creates and emails a Trello card assignment report at a button click.
Add a module
Since our scripts our written in Python, we have access to the entire universe of 3rd party modules through pip. Let's add the pystache
so that we can easily setup templates for our reporting email.
- Click Add+ next to Modules in the right sidebar.
- Search for your module, in this case pystache.
- Click the module name, and wait for your script to finish importing.
- Type
import pystache
at the top of your script and Save.
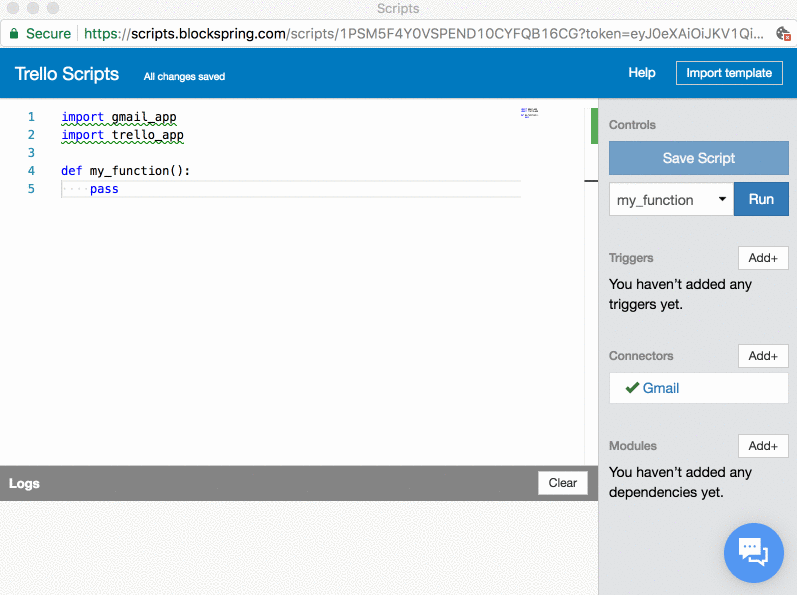
Having access to all modules over pip opens the door to many usecases for your script, including fun things with templating, machine learning, visualizations, scraping, and natural language processing.
ENABLE GMAIL
Now we'll want to add the Gmail connector to our script. Usually, integrating with another app requires setting up OAuth or getting an API token. Blockspring Scripts handles this so you can start scripting with Gmail in seconds.
- Click Add+ next to Connectors in the right sidebar.
- Choose your connector, in this case Gmail .
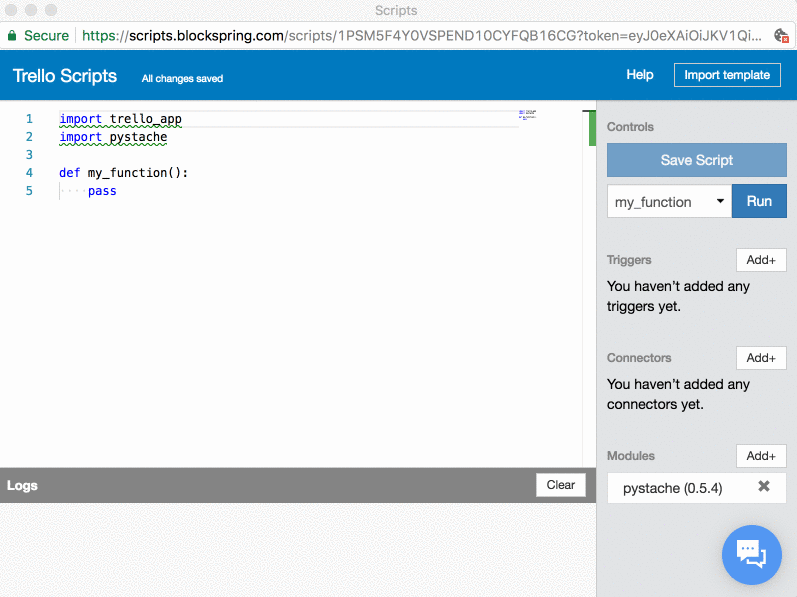
Alert - "This app isn't verified"
While in beta, Blockspring Scripts will be undergoing Google's verification process. You may notice this message from time to time as we add more connectors.
You'll notice the editor automatically handles OAuth/authentication with Google, and imports gmail_app
. You're now able to script with Sheets, just like you do with Trello.
Email Report
Since we have the templating module and Gmail integrated in our script, we can now start emailing a report. Paste in the following code into your script.
import gmail_app
import trello_app
import pystache
# SETUP 1: Sign in with Gmail.
# SETUP 2: Enter an email address below.
email = "ENTER_EMAIL_TO_SEND_REPORT_TO"
def send_report():
# Get our Trello board, members, and cards.
board = trello_app.get_current_board()
members = board.get_members()
cards = board.get_cards()
# For each member, find the number of cards they're assigned to.
member_counts = []
for member in members:
card_count = 0
for card in cards:
if member.get_field("id") in card.get_field("idMembers"):
card_count += 1
member_counts.append({ "person_name": member.get_field("fullName"), "card_count": card_count })
# Setup our email template
template = """
It's time for your Trello report! According to our calculations, {{board_name}} has {{card_count}} cards. Here's how they're assigned:
{{ #counts }}
- {{person_name}}: {{card_count}}
{{/counts}}
"""
# Populate the template with our member_counts data
message = pystache.render(template, {
"board_name": board.get_name(),
"card_count": len(cards),
"counts": member_counts
})
# Send repot using Gmail
gmail_app.send_message(message, email, subject="Trello Update")
Since we've already signed in with Gmail, the next step is to enter your email address in line 7.
Finally, let's run our send_report
. However, instead of using the test button in the editor, let's go back to Trello.
- Hover over the Blockspring leaf on your Trello board,
- Click the send_report menu option.
Check out your email - it has our report!
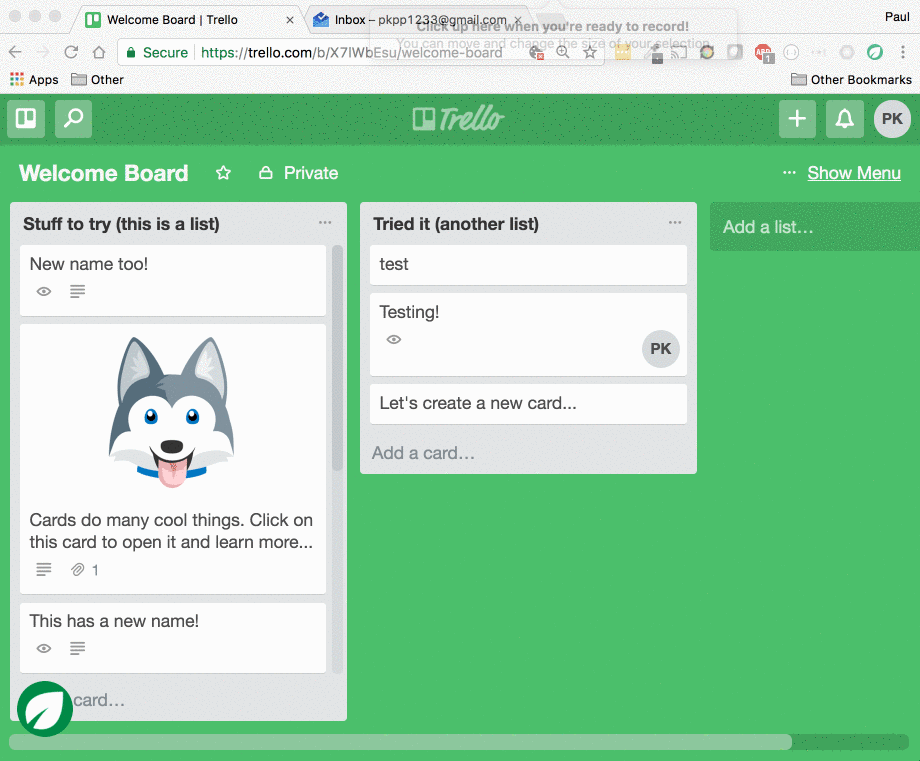
Need Help?
If you run into any issues, or what to chat with others, connect to the Blockspring community right from the Scripts editor.
Updated less than a minute ago