Tutorial: Sidebar Form
Let's learn how to give your script a front-end. In this case it's a sidebar form.
Usecase: Count Cards by Assignee
Most apps don't have a good way to generate quick reports. In this tutorial, we'll write a script that gives a front-end to our count cards function. Specifically, we'll enter a member's email and see how many cards they've been assigned.
Build our sidebar form
First thing is first - let's write a function that opens a sidebar in Trello, with a preliminary form.
import trello_app
def card_report():
# build form
return {
"_type": "form",
"_title": "Card Report",
"_inputs": [{
"name": "username",
"type": "text"
}],
"_on_submit": "count_cards_"
}
Now go back to Trello, and run the card_report
App Button.
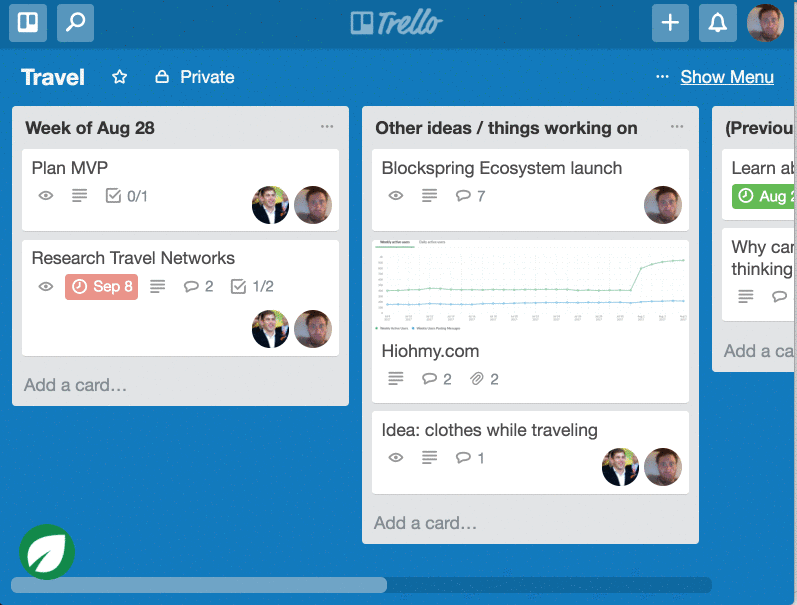
Clicking the App Button opens a sidebar with the form you specified in your script. Let's dig into the script itself.
def card_report():
# build form
return {
### _type tells us what type of UI to open. So far the only option is form.
"_type": "form",
### _title sets the title of the form sidebar.
"_title": "Card Report",
### _inputs determines what types of inputs to generate for your form. The name will be pased into your code and shown as a label. The type can be text or textarea for now.
"_inputs": [{
"name": "username",
"type": "text"
}],
### _on_submit determines what function in your script to call when they submit the form.
"_on_submit": "count_cards_"
}
So by editing that configuration, we can generate our own custom forms.
When We Submit...
You may have noticed we set count_cards_
to be the function to call when the user submits our form. Let's create our count cards function.
import trello_app
def count_cards_():
# get member from form by username
username = trello_app.get_request().get("username")
board = trello_app.get_current_board()
member = next((x for x in board.get_members() if x.get_field("username") == username), None)
# catch error if not a real member
if not(member):
return "Could not find %s" % username
# count cards
cards = board.get_cards(filter="open")
member_cards = list(filter(lambda x: member.get_field("id") in x.get_field("idMembers"), cards))
return "%s open cards for %s" % (len(member_cards), username)
def card_report():
# build form
return {
"_type": "form",
"_title": "Card Report",
"_inputs": [{
"name": "username",
"type": "text"
}],
"_on_submit": "count_cards_"
}
The most important concept to this function is .get_request()
. This lets you retrieve the form response as a dictionary/hash. Since we named the form input username
, that's the variable we'll pull out of get_request().
The rest of the function is fairly intuitive. Given the member, it counts their boards and returns a text response. Let's see the sidebar form in action.
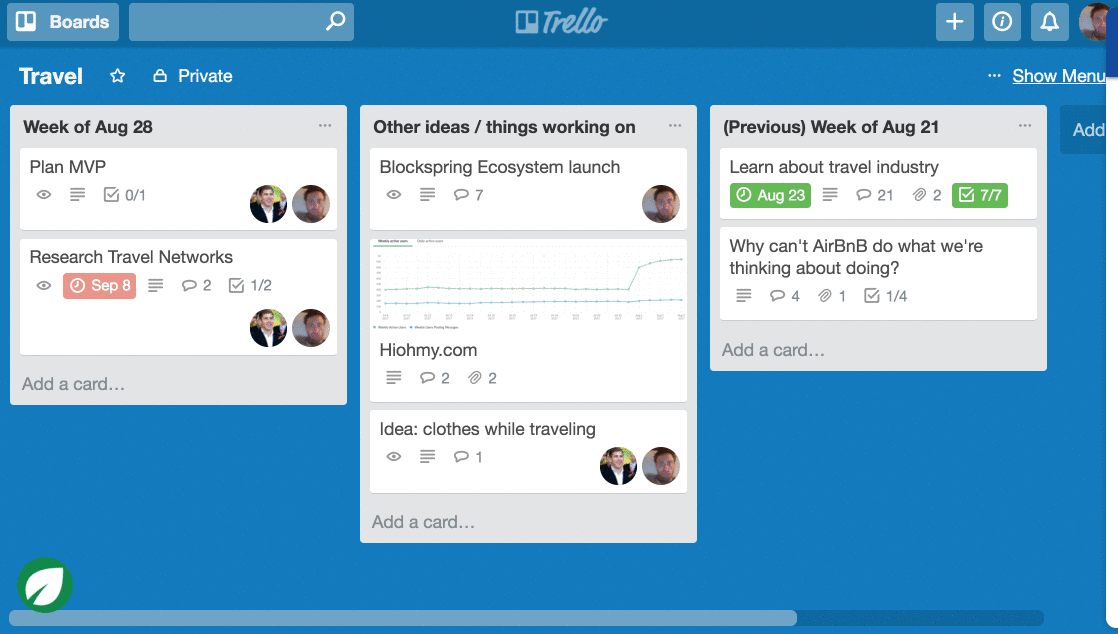
Need Help?
If you run into any issues, or what to chat with others, connect to the Blockspring community right from the Scripts editor.
Updated less than a minute ago